Understanding the Flyweight Pattern
Fly Architecture architecture, also known as flyweight architecture, is a design pattern that focuses on optimizing memory usage by sharing common data among multiple objects. This pattern is particularly useful in scenarios where a large number of similar objects need to be managed efficiently. It helps to minimize resource consumption and improve performance, especially in environments with limited memory resources.
The flyweight pattern separates the representation of an object from its state. It allows multiple instances of an object to share the same data while maintaining unique identities and behaviors. This is achieved through the use of two key components:
- Flyweight Objects: These are the shared instances that contain intrinsic state, which is the part of the object that remains constant and can be shared across multiple instances.
- Context Objects: These represent the extrinsic state, which is the variable part of the object that can change from one instance to another. This state is maintained outside of the flyweight objects.
Key Concepts
- Intrinsic vs. Extrinsic State:
- Intrinsic State: The data that is shared among all flyweight objects. For example, in a graphics application, the color and texture of a tree can be intrinsic, as multiple trees may share the same color and texture.
- Extrinsic State: The data that is unique to each instance. In the tree example, the position of each tree in a landscape would be extrinsic.
- Factory Method: A factory class is typically used to create and manage flyweight objects. It ensures that existing objects are reused instead of creating new ones, thereby optimizing resource usage.
Advantages of Fly Architecture Fly Architecture:
- Reduced Memory Usage: By sharing common data, applications can significantly reduce the number of objects in memory, leading to lower memory consumption.
- Improved Performance: With fewer objects to manage, applications can benefit from enhanced performance, especially in environments where object creation and destruction are costly operations.
- Scalability: Fly architecture allows applications to scale more effectively by managing a larger number of similar objects without a proportional increase in resource consumption.
Use Cases
- Graphics Rendering: In computer graphics, flyweight objects can represent graphical entities like sprites or shapes. By sharing properties such as color and texture, the application can render numerous entities without consuming excessive memory.
- Text Formatting: In text editors, the flyweight pattern can be employed to manage font styles and sizes efficiently. Each character can be represented as a flyweight object that shares the same font properties.
- Game Development: Many games require numerous similar objects (like bullets or enemies). The flyweight pattern allows these objects to share properties such as behavior and appearance, optimizing resource usage.
Implementing Fly Architecture
Here’s a basic implementation of the flyweight pattern in Python:
pythonCopy codeclass Flyweight:
def __init__(self, intrinsic_state):
self.intrinsic_state = intrinsic_state
def operation(self, extrinsic_state):
print(f"Intrinsic State: {self.intrinsic_state}, Extrinsic State: {extrinsic_state}")
class FlyweightFactory:
def __init__(self):
self.flyweights = {}
def get_flyweight(self, intrinsic_state):
if intrinsic_state not in self.flyweights:
self.flyweights[intrinsic_state] = Flyweight(intrinsic_state)
return self.flyweights[intrinsic_state]
# Client code
factory = FlyweightFactory()
flyweight1 = factory.get_flyweight("SharedState1")
flyweight1.operation("UniqueState1")
flyweight2 = factory.get_flyweight("SharedState1")
flyweight2.operation("UniqueState2")
assert flyweight1 is flyweight2 # True, both refer to the same object
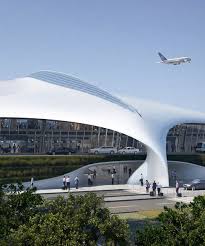
Conclusion
Fly architecture is a powerful design pattern that addresses memory efficiency in software development. By leveraging shared data and separating intrinsic and extrinsic states, developers can create applications that perform better and use resources more effectively. This pattern is particularly beneficial in scenarios involving large numbers of similar objects, making it a staple in fields like gaming, graphics, and text processing.
When considering architecture for a project, integrating the flyweight pattern can lead to significant performance improvements and reduced resource consumption, ultimately resulting in a more efficient and scalable application.
4o mini
You said:
please extend more words
ChatGPT said:
ChatGPT
Fly Architecture: A Comprehensive Overview
Introduction
Fly architecture, commonly known as the flyweight pattern, is a structural design pattern that optimizes resource management in software development by reducing memory usage. This pattern is particularly relevant when applications need to create and manage a large number of similar objects. By sharing common data, flyweight architecture allows for efficient memory use and improved performance, making it an invaluable approach in resource-constrained environments.
Understanding the Flyweight Pattern
At its core, the flyweight pattern aims to minimize memory consumption by separating the intrinsic state of an object—data that remains constant and can be shared— from its extrinsic state, which varies between instances. This distinction allows multiple instances of a class to coexist without redundant data, enabling applications to handle large volumes of similar objects efficiently.
Key Components
- Flyweight Objects: These are the core instances that contain intrinsic state. Each flyweight object represents shared data, which can be reused by different contexts. This shared nature is what sets the flyweight pattern apart from traditional object-oriented designs.
- Context Objects: These represent the extrinsic state, which is unique to each instance of the flyweight. While the flyweight object retains shared properties, the context holds data that varies, such as position, size, or any other unique attribute relevant to a particular instance.
Intrinsic vs. Extrinsic State
- Intrinsic State: This is the data that can be shared across multiple flyweight instances. For example, in a graphic application, the color and texture of a tree can be intrinsic because many trees may have the same appearance.
- Extrinsic State: This is the data that must be passed to the flyweight object when an operation is performed. In the tree example, the position of each tree in a landscape would be extrinsic, varying from one instance to another.
Advantages of Fly Architecture
Implementing the flyweight pattern offers several benefits:
- Reduced Memory Usage: By sharing intrinsic states among multiple objects, applications can significantly decrease the number of instances in memory. This is particularly crucial in scenarios where memory resources are limited, such as in mobile applications or embedded systems.
- Improved Performance: The overhead of object creation and management can be substantial. By using fewer objects, the flyweight pattern reduces the associated overhead, allowing for quicker instantiation and destruction of objects. This leads to smoother application performance, especially in scenarios involving frequent object interactions.
- Enhanced Scalability: Fly architecture allows applications to scale without a corresponding increase in resource consumption. This is particularly useful in high-demand environments, such as gaming or large-scale web applications, where managing a large number of similar objects efficiently is crucial.
- Separation of Concerns: By decoupling intrinsic and extrinsic states, the flyweight pattern promotes cleaner code architecture. This separation allows for easier maintenance and testing, as changes to the extrinsic state do not impact the shared intrinsic state.
Use Cases
The flyweight pattern is applicable in various domains, where memory optimization and performance are critical:
- Graphics Rendering: In computer graphics, flyweight objects can represent graphical entities like sprites, shapes, or characters. For instance, in a game with thousands of similar trees, using flyweight objects to share properties such as color and texture reduces memory overhead while rendering.
- Text Formatting: Text editors can employ the flyweight pattern to manage font styles, sizes, and other properties efficiently. Each character can be a flyweight object that shares common font properties, with the position and styling being extrinsic, allowing for streamlined text rendering.
- Game Development: Many games require numerous similar objects, such as bullets, enemies, or terrain features. By implementing flyweight architecture, these objects can share common characteristics while maintaining unique properties, leading to optimized performance.
- Web Development: In web applications with large datasets, the flyweight pattern can be used to manage UI components or data representations that share common styles or behaviors. This can reduce the load on the browser’s memory, enhancing user experience.
Implementing Fly Architecture
Implementing the flyweight pattern typically involves creating a factory to manage the lifecycle of flyweight objects. Here’s a more detailed implementation in Python to illustrate this concept:
pythonCopy codeclass Flyweight:
def __init__(self, intrinsic_state):
self.intrinsic_state = intrinsic_state
def operation(self, extrinsic_state):
print(f"Intrinsic State: {self.intrinsic_state}, Extrinsic State: {extrinsic_state}")
class FlyweightFactory:
def __init__(self):
self.flyweights = {}
def get_flyweight(self, intrinsic_state):
if intrinsic_state not in self.flyweights:
self.flyweights[intrinsic_state] = Flyweight(intrinsic_state)
return self.flyweights[intrinsic_state]
# Client code
factory = FlyweightFactory()
# Create shared flyweight instances
flyweight1 = factory.get_flyweight("SharedState1")
flyweight1.operation("UniqueState1")
flyweight2 = factory.get_flyweight("SharedState1")
flyweight2.operation("UniqueState2")
# Both flyweight1 and flyweight2 refer to the same object
assert flyweight1 is flyweight2 # True
In this example, the Flyweight
class encapsulates the intrinsic state, while the FlyweightFactory
manages the creation and retrieval of flyweight instances, ensuring that duplicate intrinsic states are not created. This design allows for efficient memory use and simplified management of shared objects.
Conclusion
Fly architecture is a powerful design pattern that significantly enhances memory efficiency and performance in software applications. By utilizing shared data and separating intrinsic from extrinsic states, developers can create applications capable of handling large volumes of similar objects without compromising resource consumption.
This pattern is particularly beneficial in scenarios involving graphics rendering, text processing, game development, and web applications, where the need for efficient memory management is paramount. By adopting flyweight architecture, developers can ensure that their applications are not only performant but also scalable, maintaining responsiveness even under high demand.
In summary, incorporating the flyweight pattern into application design can lead to a cleaner, more efficient codebase, enabling developers to focus on functionality while minimizing resource overhead. As software systems continue to evolve, the principles of fly architecture will remain relevant, providing a framework for optimizing resource management and enhancing application performance. For more detail please visit techwebinsights.com